- Sentinel Controlled While Loop C++
- Sentinel Controlled Loop Example
- C++ Sentinel Value
- Sentinel Controlled While Loop C++ Loop
In a sentinel-controlled while loop, the body of the loop continues to execute until the EOF symbol is read. The C Language Loops 2 Loops - Struble Loops! While Loop (Example)! Recall from our Giving Change Algorithm 1 2.2 If the value of changeis = 100, then perform the following steps. 2.2.1 Add 1 to the value of dollars. Sentinel Controlled Loop.
In computer programming, a sentinel value (also referred to as a flag value, trip value, rogue value, signal value, or dummy data)[1] is a special value in the context of an algorithm which uses its presence as a condition of termination, typically in a loop or recursive algorithm.
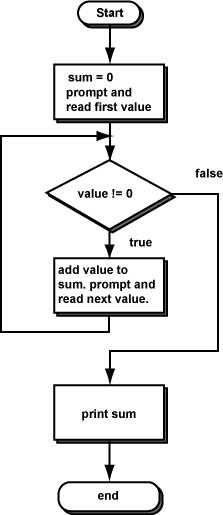
The sentinel value is a form of in-band data that makes it possible to detect the end of the data when no out-of-band data (such as an explicit size indication) is provided. The value should be selected in such a way that it is guaranteed to be distinct from all legal data values since otherwise, the presence of such values would prematurely signal the end of the data (the semipredicate problem). Ithaca model 600 review. A sentinel value is sometimes known as an 'Elephant in Cairo,' due to a joke where this is used as a physical sentinel. In safe languages, most sentinel values could be replaced with option types, which enforce explicit handling of the exceptional case.
Examples[edit]
Some examples of common sentinel values and their uses:
- Null character for indicating the end of a null-terminated string
- Null pointer for indicating the end of a linked list or a tree.
- A set most significant bit in a stream of equally spaced data values, for example, a set 8th bit in a stream of 7-bit ASCII characters stored in 8-bit bytes indicating a special property (like inverse video, boldface or italics) or the end of the stream
- A negative integer for indicating the end of a sequence of non-negative integers
Variants[edit]
A related practice, used in slightly different circumstances, is to place some specific value at the end of the data, in order to avoid the need for an explicit test for termination in some processing loop, because the value will trigger termination by the tests already present for other reasons. Unlike the above uses, this is not how the data is naturally stored or processed, but is instead an optimization, compared to the straightforward algorithm that checks for termination. This is typically used in searching.[2][3]
For instance, when searching for a particular value in an unsorted list, every element will be compared against this value, with the loop terminating when equality is found; however, to deal with the case that the value should be absent, one must also test after each step for having completed the search unsuccessfully. By appending the value searched for to the end of the list, an unsuccessful search is no longer possible, and no explicit termination test is required in the inner loop; afterward, one must still decide whether a true match was found, but this test needs to be performed only once rather than at each iteration.[4]Knuth calls the value so placed at the end of the data, a dummy value rather than a sentinel.
Examples[edit]
Array[edit]
For example, if searching for a value in an array in C, a straightforward implementation is as follows; note the use of a negative number (invalid index) to solve the semipredicate problem of returning 'no result':
However, this does two tests at each iteration of the loop: whether the value has been found and whether the end of the array has been reached. This latter test is what is avoided by using a sentinel value. Assuming the array can be extended by one element (without memory allocation or cleanup; this is more realistic for a linked list, as below), this can be rewritten as:
The test for i < len
is still present, but it has been moved outside the loop, which now contains only a single test (for the value), and is guaranteed to terminate due to the sentinel value. There is a single check on termination if the sentinel value has been hit, which replaces a test for each iteration.
It is also possible to temporarily replace the last element of the array by a sentinel and handle it, especially if it is reached: Waves cla vocals plugin torrent.
See also[edit]
References[edit]
- ^Knuth, Donald (1973). The Art of Computer Programming, Volume 1: Fundamental Algorithms (second edition). Addison-Wesley. pp. 213–214, also p. 631. ISBN0-201-03809-9.
- ^Mehlhorn, Kurt; Sanders, Peter (2008). Algorithms and Data Structures: The Basic Toolbox 3 Representing Sequences by Arrays and Linked Lists(PDF). Springer. ISBN978-3-540-77977-3. p. 63
- ^McConnell, Steve (2004). Code Complete (2nd ed.). Redmond: Microsoft Press. p. 621. ISBN0-7356-1967-0.
- ^Knuth, Donald (1973). The Art of Computer Programming, Volume 3: Sorting and searching. Addison-Wesley. p. 395. ISBN0-201-03803-X.
In programming languages – while coding a program, sometimes it is necessary to repeat a set of statements several times (Looping Control Structures ). A way to repeat statements is to type the same statements in the program over and over. For example, if you want to repeat some statements 100 times, you type the same statements 100 times in the program. However, this solution of repeating statements is impractical, if not impossible. Fortunately, there is a better way to repeat a set of statements.
As noted earlier, C++ has three repetition, or looping control structures that allow you to repeat a set of statements until certain conditions are met.
Note: The variable that controls the loop is called loop control variable (LCV)
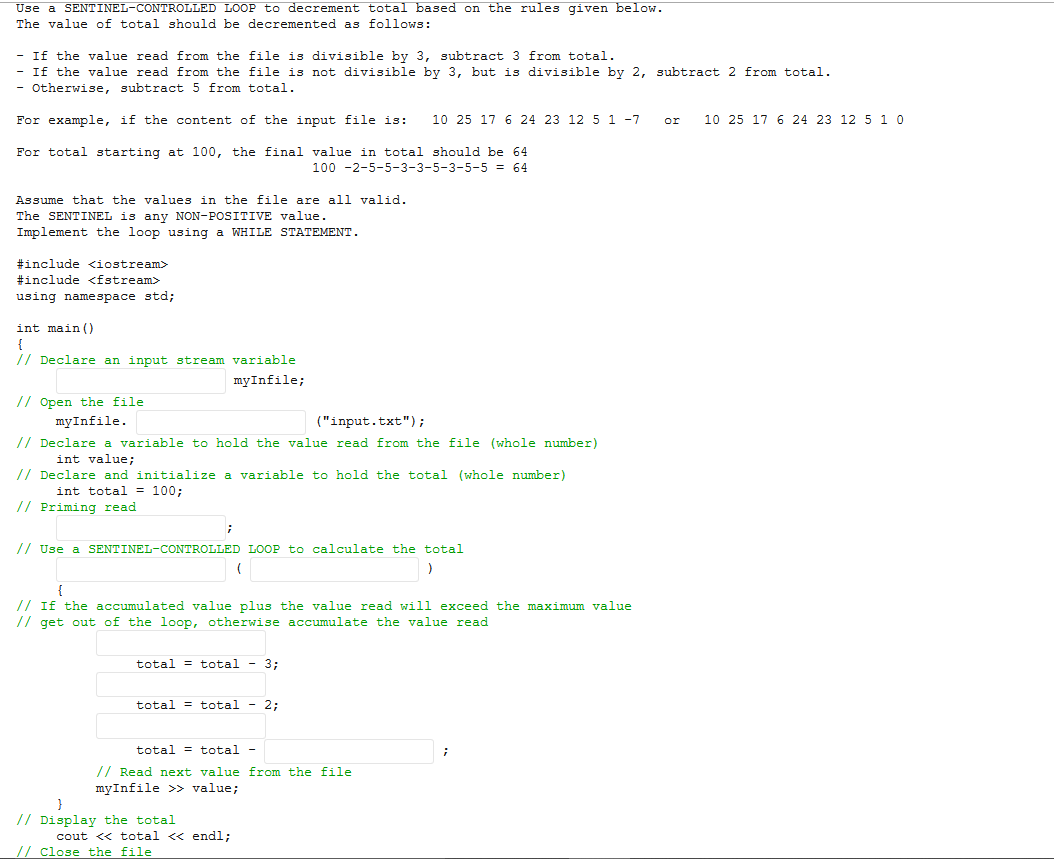
The first loop that we’re going to discuss about is while loop. The general form of while loop is:
In C++, whileis a reserved word. Of course, the statement can be either a simple or compound statement. The expression acts as a decision maker and is usually a logical expression. The statement is called the body of the loop. Note that the parentheses around the expression are part of the syntax.
In Line 1, the variable x is set to 0. The expression in the while statement (in Line 2), x <= 20, is evaluated. Because the expression x <= 20 evaluates to true, the body of the while loop executes next. The body of the while loop consists of the statements in Lines 3 and 4. The statement in Line 3 outputs the value of x, which is 0. The statement in Line 4 changes the value of x to 5. After executing the statements in Lines 3 and 4, the expression in the while loop (Line 2) is evaluated again. Because x is 5, the expression x <= 20 evaluates to true and the body of the while loop executes again. This process of evaluating the expression and executing the body of the while loop continues until the expression, x <= 20 (in Line 2), no longer evaluates to true.
Kinds of while loops are: counter-controlled, flag-controlled, sentinel-controlled, EOF-controlled whileloops and etc.
The general form of for loop is:
The initial statement, loop condition, and update statement (calledfor loop control statements) enclosed within the parentheses control the body (statement) of the for statement.
The for loop executes as follows:
- The initial statement executes.
- The loop condition is evaluated. If the loop condition evaluates to true:
- Execute the for loop statement.
- Execute the update statement (the third expression in the parentheses).
- Repeat Step 2 until the loop condition evaluates to false.
The initial statement usually initializes a variable (called the for loop control, or forindexed, variable).
Sentinel Controlled While Loop C++
In C++, foris a reserved word.
Concentrate on the example bellow:
The initial statement, x = 0;, initializes the int variable x to 0. Next, the loop condition, x<10, is evaluated. Because 0<10 is true, the print statement executes and outputs 0. The update statement, x++, then executes, which sets the value of x to 1. Once again, the loop condition is evaluated, which is still true, and so on. When x becomes 10, the loop condition evaluates to false, the for loop terminates, and the statement following the for loop executes.
This section describes the third type of looping or repetition structure, called a do…while loop. The general form of a do…while statement is as follows:
Sentinel Controlled Loop Example
The statement executes first, and then the expression is evaluated. If the expression evaluates to true, the statement executes again. As long as the expression in a do…while statement is true, the statement executes. To avoid an infinite loop, you must, once again, make sure that the loop body contains a statement that ultimately makes the expressionfalse and assures that it exits properly.
The output of the code is:
0 5 10 15 20
After 20 output, the statement:
x = x +5;
changes the value of x to 25 and so x <=20 becomes false, which halts the loop.
C++ Sentinel Value
- In a while and for loop, the loop condition is evaluated before executing the body of the loop. Therefore, while and for loops are called pretest loops. On the other hand, the loop condition in a do…while loop is evaluated after executing the body of the loop. Therefore, do…while loops are called post-test loops. Because the while and for loops both have entry conditions, these loops may never activate. The do…while loop, on the other hand, has an exit condition and therefore always executes the statement at least once. Looping Control Structures
Sentinel Controlled While Loop C++ Loop
“The end of Looping Control Structures in C++.
